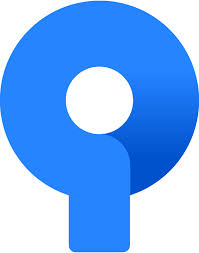
Just a quick post (I know, it’s been a while).
Was having a heck of a time getting SourceTree to push to GitHub via ssh and oauth. It would just hang at pushing and never complete. I’d saved my pubkey from SourceTree into Github, so the trust relationship should have been there.
Finally went into the local repo in terminal, and did a “git push”. That then grabbed the SSH key from GitHub and prompted to save to known hosts, then pushed.
Went back into SourceTree after making a simple change to a file, and was able to successfully Commit and push to Github. So for whatever reason, the issue was SourceTree wasn’t prompting to save the ssh key so it was just getting stuck waiting for confirmation.
Hopefully this helps someone!